Custom attribute with AureliaJS and Moment
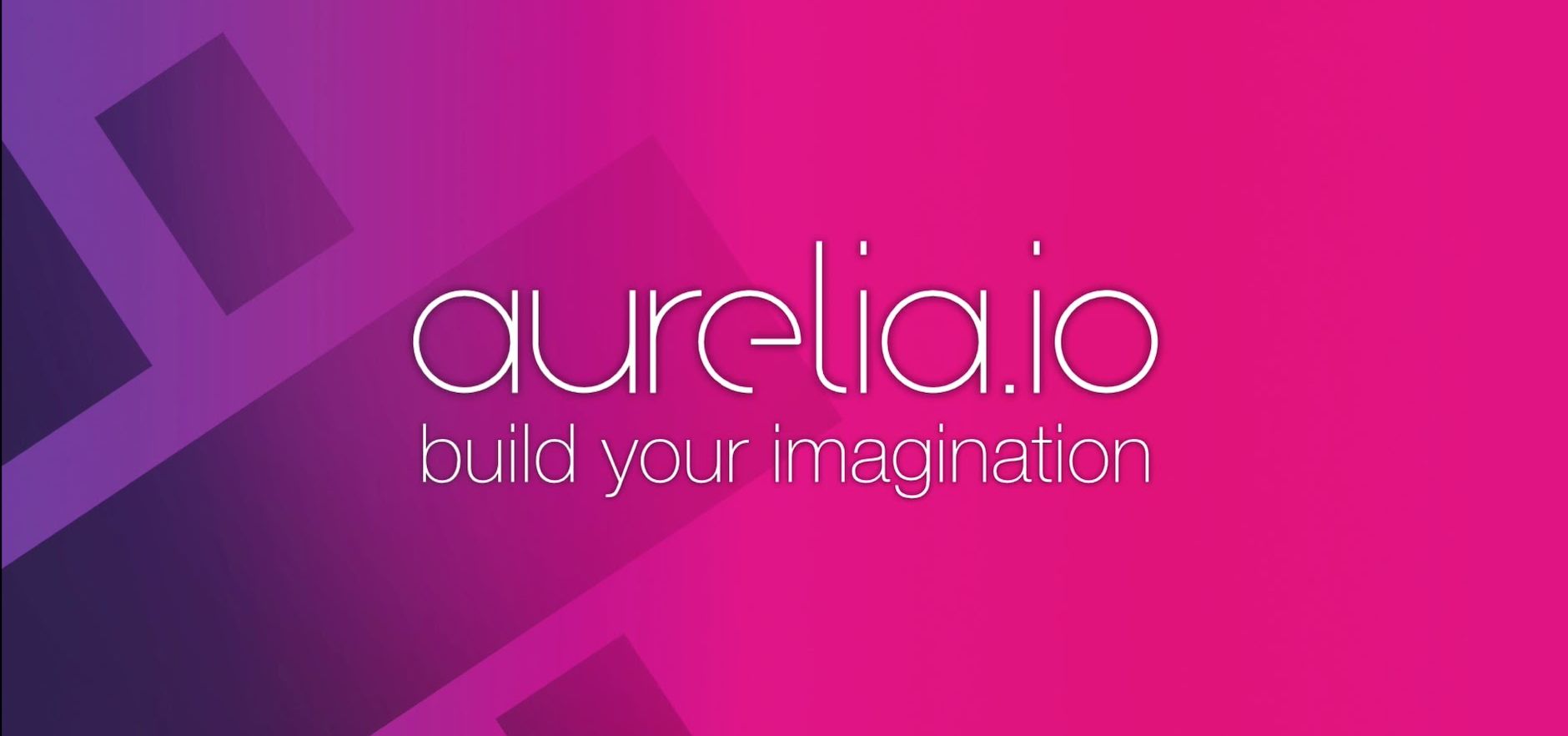
Today I need to create a simple custom component/attribute to handle some formatting of dates on-the-fly in the html.
Working with AureliaJS is really easy to create the custom components / attributes. Also Moment is really awesome for date formatting.
What I need is something that can pickup the date inside the element and format it the way I specify.
in the html I want to have something like this:
|
|
The format being passed to article.created_at
is 2016–06–19T22:42:38Z
First we need to create a simple file that is going to be our custom attribute
|
|
The code is pretty simple, we just import what we need: inject, bindable and customAttribute, and moment. Then we specify the name of our attribute, and the property that we need to specify the format of the date.
In the bind() we just grab the innerHTML of our element and use moment to convert it, using the format that we pass. Dont forget to install moment by for example, using jspm:
|
|
To use the custom attribute you have 2 ways: either before the place where you want to use it you call require on the path to the custom attribute, like this:
|
|
Or if you need to use it in multiple places, you need to make it load automatically by defining it in the globalResources of your Aurelia app.
|
|
In the end, I get a properly formatted datetime: 2016–06–19 23:42