Basic Swift, part 3 - Optionals
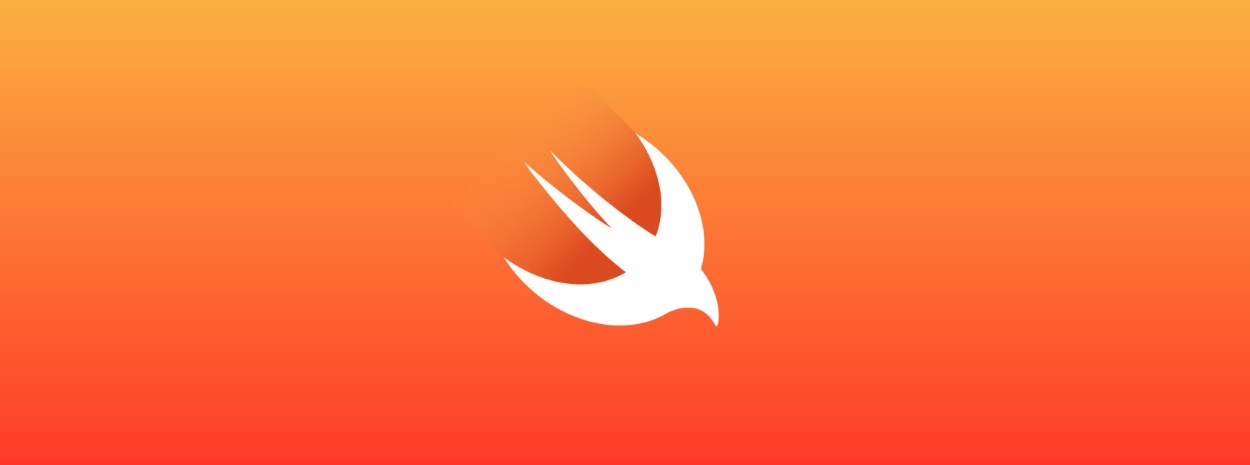
Swift Optionals
Optional
is an instance variable that may or may not have a value.
In Swift is not possible to use a variable that is empty. Variables cannot be nil
(will crash program if it happens).
So by setting a variable to be optional, Swift is creating a bubble around that variable to protect it from ever being nil.
So if there is a variable that is possible that in some point in time will be nil, we have to declare it has optional, like this:
|
|
Forced Unwrap
We can use forced unwrap
to get the value, but we must be sure that it has a value
|
|
NOTE: Optionals cannot be constants. You need to use
var
to define them.
By declaring myBankAccountBalance
as an optional variable, to use it it must be unwrapped
.
Optional binding
But what happens if we are not sure if we have a value in the variable?
In this case we have to use Optional binding
:
|
|
unwrappedBalance
will only exists inside of that code block.
Implicitly unwrapped
We can also do an Implicitly unwrapped options
by using the !
on creation. This way:
|
|